Attaching additional responsibilities to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality.
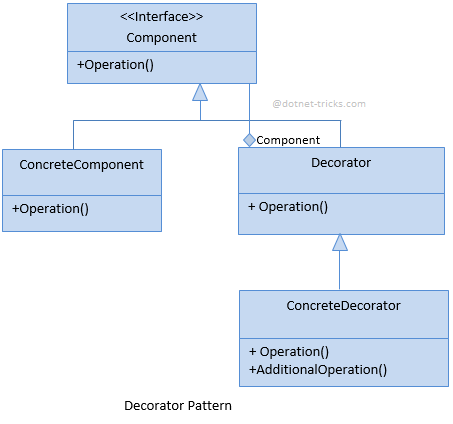
Code :-
//Component
namespace DecoratorDemo.Component
{
public interface IHome
{
string Make { get; }
double GetPrice();
}
}
//Concrete Component
public class MyHome : Component.IHome
{
public string Make {
get
{
return "MyHome";
}
}
public double GetPrice()
{
return 8000000;
}
}
//Decorator
public abstract class HomeDecorator : IHome
{
private readonly IHome home;
public HomeDecorator(IHome home)
{
this.home = home;
}
public string Make
{
get
{
return "MyHome";
}
}
public double GetPrice()
{
return home.GetPrice();
}
public abstract double GetDiscountPrice();
}
// Concrete Decorator
public class OfferPrice : HomeDecorator
{
public OfferPrice(IHome home) : base(home)
{
}
public override double GetDiscountPrice()
{
return .8 * base.GetPrice();
}
}
// Execution
class Program
{
static void Main(string[] args)
{
IHome home = new MyHome();
HomeDecorator decorator = new OfferPrice(home);
Console.WriteLine(string.Format("Make : {0} Price {1} DiscountPrice {2}", decorator.Make, decorator.GetPrice(), decorator.GetDiscountPrice()));
}
}
OutPut
Make : MyHome Price 8000000 DiscountPrice 6400000
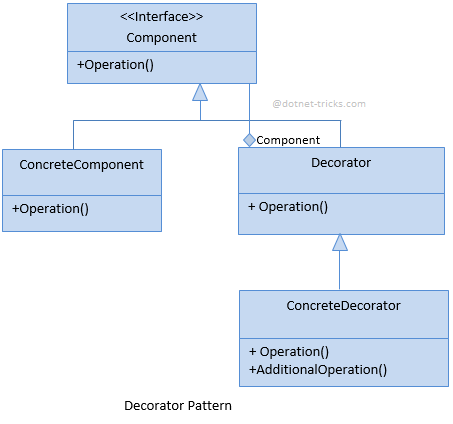
The classes and objects participating in this pattern are:
- Component
- defines the interface for objects that can have responsibilities added to them dynamically.
- ConcreteComponent
- defines an object to which additional responsibilities can be attached.
- Decorator
- maintains a reference to a Component object and defines an interface that conforms to Component's interface.
- ConcreteDecorator
- adds responsibilities to the component.
Code :-
//Component
namespace DecoratorDemo.Component
{
public interface IHome
{
string Make { get; }
double GetPrice();
}
}
//Concrete Component
public class MyHome : Component.IHome
{
public string Make {
get
{
return "MyHome";
}
}
public double GetPrice()
{
return 8000000;
}
}
//Decorator
public abstract class HomeDecorator : IHome
{
private readonly IHome home;
public HomeDecorator(IHome home)
{
this.home = home;
}
public string Make
{
get
{
return "MyHome";
}
}
public double GetPrice()
{
return home.GetPrice();
}
public abstract double GetDiscountPrice();
}
// Concrete Decorator
public class OfferPrice : HomeDecorator
{
public OfferPrice(IHome home) : base(home)
{
}
public override double GetDiscountPrice()
{
return .8 * base.GetPrice();
}
}
// Execution
class Program
{
static void Main(string[] args)
{
IHome home = new MyHome();
HomeDecorator decorator = new OfferPrice(home);
Console.WriteLine(string.Format("Make : {0} Price {1} DiscountPrice {2}", decorator.Make, decorator.GetPrice(), decorator.GetDiscountPrice()));
}
}
OutPut
Make : MyHome Price 8000000 DiscountPrice 6400000
No comments:
Post a Comment